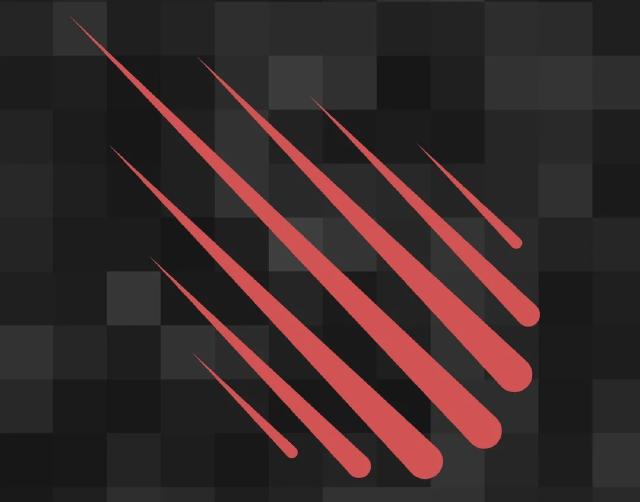
Optimistic UI in Meteor.js 3: A Component-Based Approach with Meteor.callAsync
Understanding Optimistic UI
Optimistic UI is a design pattern that prioritizes user experience by immediately updating the UI after a user action, even before the server confirms the operation. This creates a perception of instant responsiveness, enhancing user satisfaction. Meteor.js, with its reactive nature, is well-suited for implementing optimistic UI.
Fetching Data and Initializing State
Before diving into optimistic updates, let’s establish a solid foundation for fetching data when a component mounts.
import { Meteor } from 'meteor/meteor';
import { Template } from 'meteor/templating';
import { ReactiveVar } from 'meteor/reactive-var';
Template.myComponent.onCreated(function () {
const instance = this;
instance.data = new ReactiveVar([]);
Meteor.callAsync('myCollection.getAll')
.then(result => {
instance.data.set(result);
})
.catch(error => {
console.error('Error fetching data:', error);
});
});
Template.myComponent.helpers({
data() {
return Template.instance().data.get();
}
});
This code demonstrates:
- Using
ReactiveVar
to manage the component’s data. - Employing
Meteor.callAsync
to fetch data asynchronously when the component mounts. - Updating the
data
ReactiveVar with the fetched data.
Implementing Optimistic UI
Let’s extend the component to incorporate optimistic updates:
import { Meteor } from 'meteor/meteor';
import { Template } from 'meteor/templating';
import { ReactiveVar } from 'meteor/reactive-var';
Template.myComponent.onCreated(function () {
// ... previous code
this.itemToEdit = new ReactiveVar(null);
});
Template.myComponent.events({
'click .edit'(event, template) {
const item = Template.currentData();
template.itemToEdit.set(item);
// Optimistic update (modify item directly in UI)
item.name = 'New Name'; // Example of an optimistic update
Meteor.callAsync('myCollection.update', item._id, { name: 'New Name' })
.then(() => {
// Successful update
})
.catch(error => {
// Handle error, revert optimistic update
item.name = item.originalName; // Assuming originalName is stored somewhere
});
},
'submit .edit-form'(event, template) {
// Handle form submission and potential additional updates
}
});
This code demonstrates:
- Using
ReactiveVar
to manage the item being edited. - Performing an optimistic update by directly modifying the item in the UI.
- Using
Meteor.callAsync
to update the item on the server. - Revert the optimistic update if the server operation fails.
Key Considerations
- Use
ReactiveVar
for efficient state management. - Employ
Meteor.callAsync
for asynchronous server interactions. - Implement robust error handling to maintain UI consistency.
- Consider using a loading state to indicate ongoing operations.
- For complex scenarios, explore using a state management library like Redux or Zustand.
By following these guidelines, you can effectively implement optimistic UI in your Meteor.js 3 components, providing a smoother and more responsive user experience.